Related topic: [link](https://community.robotshop.com/forum/t/lynxmotion-rovPS2X_ExampleRover.zip (878 Bytes) er-ps2-controller-arduino-code/71804).
I’m using the sample code for the Ps2 remote with the rover. It is reading commands from the controller but only the left or the right motors will move at once, meaning it basically will just spin in a circle, one way or the other. Does anyone have any insights on getting the rover to move both left and right motors at the same time?
When I call servo.write(90) I get movement, which is supposed to be the neutral setting. When I call servo.write(0) I get no movement. I’m not sure how to get basic move forward, then stop functionality.
2 Likes
Hey @Cambone
I’ll need some extra info to assist you! See below:
- Can you link to the exact version of the code you are currently using? (if you’ve modified it, you can attach it here as a .zip)
- Please offer some details on the exact wiring you are using (arduous-compatible board, motor driver, etc.).
- Any other relevant info you have that could help!
Sincerely,
1 Like
If only one motor turns, that’s the mixing on the Sabertooth. Assuming you purchased the kit below, it came with the Sabertooth 2x12.
Open the documentation included with that guide, and then the Quick Start Guide within that ZIP file. Under Options, you’ll see the DIP switch configurations possible. It sounds like your issue is “Mixing Mode”.
Also, ensure you have it wired like this with the two center (red) pins from the Sabertooth removed before being connected to the BotBoarduino (as indicated in the manual):
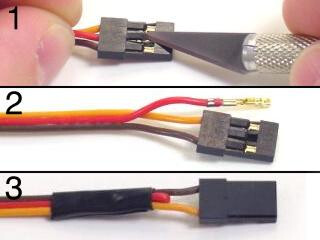
1 Like
I’ve followed the assembly directions very closely. I’ve double checked the Sabertooth settings and the wiring. I was able to get the rover to move forward, and then turn right, but it gets stuck once turning right and will not stop. Here is my code. I’ve also confirmed that the motors are reading the values I’m sending in, but the motors don’t seem to be responding as specified in the Servo.h documentation.
//*********************************************************************************
// www.Lynxmotion.com
// Basic code for 2WD rover using continuous rotation servos, controlled via PS2
// Right now, the library does NOT support hot pluggable controllers, meaning
// you must always either restart your Arduino after you connect the controller,
// or call config_gamepad(pins) again after connecting the controller.
//*********************************************************************************
#include <PS2X_lib.h> //for v1.6
#include <Servo.h>
// create PS2 Controller Class
PS2X ps2x;
int error = 0;
byte vibrate = 0;
// create servo objects to control the servos
Servo servoleft; // left servo
Servo servoright; // right servo
#define Deadzone 10 //PS2 analog joystick Deadzone
void setup(){
Serial.begin(9600);
// Attaches servos to digital pins 3 and 4 on the BotBoarduino
servoleft.attach(3);
servoright.attach(4);
pinMode(7, INPUT);
error = ps2x.config_gamepad(9,7,6,8, true, true);
}
void loop(){
ps2x.read_gamepad(); //read controller
// This code uses the colored buttons on the right side of the joystick
//Forward
if(ps2x.Button(PSB_RED)) {
servoleft.write(0);
servoright.write(90);
Serial.println(servoleft.read());
Serial.println(servoright.read());
}
//Turn right
else if(ps2x.Button(PSB_PINK)){
servoleft.write(90);
servoright.write(90);
Serial.println(servoleft.read());
Serial.println(servoright.read());
}
//Servo left and right must be set to 0 otherwise rover will move on startup
else {
servoleft.write(0); // Adjust these values if the servos still move slightly
servoright.write(0);
Serial.println(servoleft.read());
Serial.println(servoright.read());
}
delay(50);
}
- Can you provide a few clear photos of the connections to each board?
- Can you try the PS2 example and verify using the Arduino IDE’s serial terminal that the commands are picked up correctly?
http://www.billporter.info/2010/06/05/playstation-2-controller-arduino-library-v1-0/
Based on the behavior, one thing which comes to mind is the batteries used in the remote may be too drained (they don’t last long and the remote needs to be turned off when not in use).
Another thought is that the motor wiring might be off. Can you pick up the rover (put the chassis on a shoebox to let the wheels spin freely) and then test, and verify the motion of all four wheels is correct? For testing, and troubleshooting it’s best to proceed like this rather than on the ground.
The commands from the controller are definitely working, as I am reading the values back from the servos in my code and writing them to the serial log. The batteries are new. I’m beginning to think I have a bad Sabertooth module.
Hmm… so the commands show up via the serial monitor correctly, which means everything between the remote to the connections to the BotBoarduino are correct.
The fact that the BotBoarduino can control the motors is also a good sign.
Based on your photo, it seems you’ve removed the red wires correctly, so there’s not a conflict in power.
Q1) Can you provide a top-down photo of the BotBoarduino (with some area around to see some wires?)
Q1) When you lift the rover off the ground and make the wheels move, do they behave as expected? Are any wheels’ directions reversed?
Q3) Triple check the connections to the motors (the two pins on each motor) and for the heck of it (with the battery removed), remove the wires from the Sabertooth (the ones going to the motors) and re-wire them just to be 100% certain there’s good contact.
The Sabertooth is normally a really high quality motor controller, but on occasion there are defects. Let’s work out for reasonable certainty that the Sabertooth’s the cause as opposed to something else, then proceed from there with an exchange.
The video seems to show that all four wheels move in the correct direction, is that what you wanted to show?
The setup looks good, and note that for testing, you might want to use VL=USB to save the 9V battery.
The pinout seems to match the code.
Did you ever connect the 5V wires from the Sabertooth to the BotBoarduino? This would cause a conflict and potentially damage the main chip, leading to erratic behavior.
DIP switch positions.
- Enable mixed mode seems fine
- Don’t need exponential
- You’re not using a Lithium based battery? What battery do you have connected?
- Don’t need ramping
- Don’t need autocal.
- Don’t need timeout
No, I never connected the 5v to the board. All wheels move in one direction but I can’t get them to stop or do anything else. The command I’m using for forward motion also makes no sense. See below, 0 to left, 90 to right? But for some reason, it works.
//Forward
if(ps2x.Button(PSB_RED)) {
servoleft.write(0);
servoright.write(90);
Here’s my wiring to the Sabertooth from the motors. The top wire terminal is slightly misaligned on the board, not sure if that has anything to do with it. I soldered the wires together going into the terminal.
There seems to be a significant amount of exposed wire around the terminals… normally the wires need to be shielded up until the screw terminals to prevent any touching which would effectively fry the board. You’ll need to correct this ASAP, and not sure if something bad may have already happened.
Do you have some experience writing code? The Sabertooth RC takes input like an RC servo, so you can use an Arduino servo example, where 1500us is “stopped” to control each channel. There’s a “servo sweep” example you might try, but modify the delay at the bottom to something like 2000 (2s) to be able to see what happens with the wheels and their direction.
Normally the products included in the A4WD kits are not problematic. Based on what you wrote it seems like the motor controller may be the issue and it seems the manufacturer of the Sabertooth (Dimension Engineering) is now involved via Zendesk and have responded. It seems the original tech who took over forgot to provide Dimension Engineering with a link to this forum thread.
We’ll close the thread here and continue via Zendesk.
This topic was transferred to internal RobotShop Support.