Hello,
I’m pretty new to Lynxmotion products and Arduino, so I apologize if this is a very trivial question.
I’m working with the LSS-HT1, and using Arduino to send it commands using a Arduino Mega 2560 and the LSS Adapter Board.
In trying to run and debug my own script, I’ve run into the issue of the LSS Communication Protocol commands being printed to the serial monitor every time I send a command to the servo. For example, this is what prints to the serial monitor when I run LSS_Sweep (example from the Arduino library):
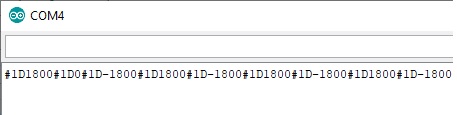
In some cases this is convenient, but for my purposes I would rather not see these commands every time. Is there a simple way to suppress this output while being able to print other data to the serial monitor?
My first thought was that it has something to do with this line:
// Initialize the LSS bus
LSS::initBus(Serial, LSS_BAUD);
But messing with this line has lead to the servo not receiving commands at all. I’ve looked at the .h file for the LSS library but haven’t been able to figure it out.
Thanks!
1 Like
Hey @ekjaynes!
It seems what is happening here is that you are using the same serial bus for both communicating with your LSS and also your serial monitor window. Many of the Arduino boards that exist (ex: Arduino Uno and many others based on the ATmega328 chip) have only one hardware serial port. Therefore, using that port (ex: Serial) will lead to everything you do on that bus being shown in the serial monitor since it is the same hardware bus that is used with the USB<>UART interface to program/communicate with the Arduino board.
There are two typical options in this case:
- Use a board that has 2 or more hardware UART peripherals, such as an Arduino Mega (ex: boards based on the ATmega2560) or a Teensy (I think version 3.0 & up have 3+ hardware ports!).
- Use a software serial port. The LSS Arduino library actually supports software serial ports so you can call the LSS::initBus function with a software serial object instead of the hardware serial one (Serial).
For software serial, you can do so easily if you are using an LSS Adapter Board with your Arduino board and LSS.
Take any example from the LSS Arduino Library, such as LSS_Sweep and modify a few key lines to match the code below.
Main changes are:
- Add #include for SoftwareSerial.h. This is needed to have the option of using it!
- Initiate the SoftwareSerial object (in my case it is called mySoftSerial) for pins 8/9. You can read more on our wiki about the SoftwareSerial access on the LSS Adapter Board, which is done by placing the communication switch in XBee mode.
- Replace the word Serial with your software serial object (in this example mySoftSerial).
/*
* Author: Sebastien Parent-Charette ([email protected])
* Version: 1.1.0
* Licence: LGPL-3.0 (GNU Lesser General Public License version 3)
*
* Desscription: Basic example of the LSS moving back and forth.
*/
#include <SoftwareSerial.h>
#include <LSS.h>
// ID set to default LSS ID = 0
#define LSS_ID (0)
#define LSS_BAUD (LSS_DefaultBaud)
// Create one LSS object
LSS myLSS = LSS(LSS_ID);
// Create a software serial port (LSS Adapter board used in XBee mode)
SoftwareSerial mySoftSerial(8, 9);
void setup()
{
// Initialize the LSS bus
LSS::initBus(mySoftSerial, LSS_BAUD);
// Initialize LSS to position 0.0 �
myLSS.move(0);
// Wait for it to get there
delay(2000);
}
Try and see if this works for you if you have a LSS Adapter Board.
Sincerely,
1 Like
I see what you’re saying about using the same serial bus for communication and the serial monitor, that makes sense! The code you included does exactly what I’m looking for.
Theoretically, if I wanted to use one of the other hardware ports with my Mega, how could I do that? Or would it just be easier to stick with the software serial port?
Thank you!
1 Like

I just noticed you were using an Arduino Mega in your other posts on our community! Yeah, stick to hardware port since they are more reliable (hardware buffering, interrupts, etc.) whereas SoftwareSerial - while very useful - requires much overhead on the CPU to process all the signals coming in and out (basically “bit-banging” the bus using the CPU)!
If you can, it is always much preferred to use hardware peripherals! They exist specifically to reduce CPU processing, overhead, etc.!
Just use Serial2, Serial3, etc. You can find more info here about serial ports on Arduino.
I hope that helps!
Sincerely,
1 Like