As I mentioned I want to
As I mentioned I want to create a 3D model of my robot to help me understand and visualize the math better. The idea is to end up with an application that besides being the brains of the robot, also visualizes the robot itself, as well as the sensor data.
Last night I was playing around with Visual Python and made this:
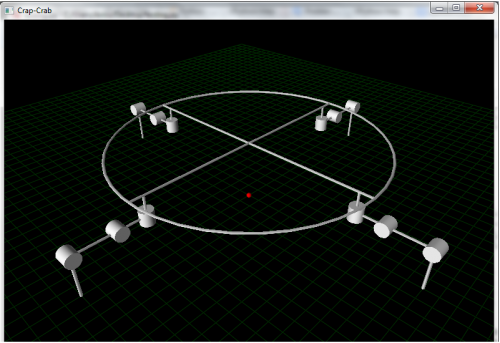
It ain't pretty :) But it isn't supposed to be. I wanted it to be as simple as possible. I didn't use modeling software, I simply draw 3D primitives (lines, cylinders, ring) onto the screen using code.
The idea is to use vectors to represent ..well.. everything. E.g. each leg segment is represented by a vector, and the leg is then drawn onto the screen based on this vector. There will also be a vector representing the axis of each servo (the cylinders). This way when I wan't to move a servo I simply use the function rotate() provided by VPython. E.g. if A is the leg segment vector, B is the servo axis vector and I want to rotate theta degrees, I'll simply write: A.rotate(theta,B). And that's it.
Also if I want to know the position of the end point (or any other point) of a leg given the servo positions (aka Forward Kinematics), it's simply a matter of adding the leg segment vectors up to that point. E.g. if A is the coxa, B the femur and C the tibia, then the simple vector sum A+B+C would give me the end point position.
As mentioned before I'll be using different frames. One for each leg, one for the robot itself and the "world" frame. As it turns out translating between these frames is actually pretty simple (if I understand it correctly), using a translation matrix:
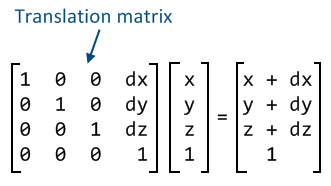
For instance the leg segment and servo positions are given relative to the leg frame, and the leg position relative to the robot frame. If I want to know the leg segment and servo positions relative to the robot frame, I simply solve this matrix with (x,y,z) being the respective positions of leg segments and servos relative to the leg frame, and (dx,dy,dz) being the position of the leg relative to the robot frame. And that should be it.
There are still several things I'm having trouble wrapping my head around though. For instance... in Forward Kinematics you always have exactly one solution. But in Inverse Kinematics you may have no solution or many/infinite solutions. Not sure how to manage this yet?!
Anyway that will be it... for now :P