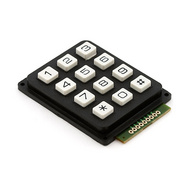
Keypad_1-0.zip (9984Bytes)
Hi, got this keypad from my local sparkfun distribuitor, but wasn't sure how to wire it up, the pins aren't in order, like on most other tutorials/examples, so here's how to have it up nd running in no time!
just wire it like this:
I know about the ''arudino'' mistake, sorry, typo :(
And run this code:
/* @file HelloKeypad.pde
|| @version 1.0
|| @author Alexander Brevig
||
|| @description
|| | Demonstrates the simplest use of the matrix Keypad library.
|| #
*/
#include <Keypad.h>
const byte ROWS = 4; //four rows
const byte COLS = 3; //three columns
char keys[ROWS][COLS] = {
{'1','2','3'},
{'4','5','6'},
{'7','8','9'},
{'#','0','*'}
};
byte rowPins[ROWS] = {5, 4, 3, 2}; //connect to the row pinouts of the keypad
byte colPins[COLS] = {8, 7, 6}; //connect to the column pinouts of the keypad
Keypad keypad = Keypad( makeKeymap(keys), rowPins, colPins, ROWS, COLS );
void setup(){
Serial.begin(9600);
}
void loop(){
char key = keypad.getKey();
if (key != NO_KEY){
Serial.println(key);
}
}
be sure to have the keypad library installed, it's attached to this post.
For more details, go here: